Booleans and Conditional logic¶
2 == 3
False
2 < 3
True
2 > 3
False
We will often use comparison operators between two variables, or between a variable and a value:
#assigning a variable
a = 2
#Applying the comparison operator: Is a equal to 2? Well yes, we just defined it!
print(a == 2)
True
b = 3
#Applying a comparison between two variables:
print(a == b)
False
The following comparison operators exist between two integer variables: Assume a = 2
and b = 3
as above.
operator | Description | Result | Example (a, b = 2, 3 ) |
---|---|---|---|
== | a equal to b | True if a has the same value as b | a == b # False |
!= | a not equal to b | True if a does NOT have the same value as b | a != b # True |
> | a greater than b | True if a is greater than b | a > b # False |
< | a less than b | True if a is less than be b | a < b # True |
>= | a greater than or equal to b | True if a is greater than or equal to b | a >= b # False |
<= | a less than or equal to b | True if a is less than or equal to b | a <= b # True |
Note: The result of a comparison is defined by the type of a and b, and the operator used.
All conditional checks must always resolve to True
or False
, or an error if the two object types are not comparable.
#try it out!
print(a > b)
print(a < b)
print(a != b)
False True True
#Just like a variable, we can also store the result of the comparison. It will be a boolean
result = a > b
print(result)
print(type(result))
False <class 'bool'>
The same operators can also be applied to two string variables.
Greater/smaller than comparisons are based on the alphanumeric sort:
print("a" == "b")
print("a" != "b")
False True
print("a" < "b")
print("a" > "b")
#also works on multi character strings:
print("carl" < "cbrl")
True False True
#Why is "cdk10" smaller than "cdk8"?
print("cdk10" < "cdk8")
True
Equality¶
More generally, we can test the equality of two variables or objects with the equality operator ==
.
Operator | Description | Example (a, b = 2, 3 ) |
---|---|---|
== | True if both sides evaluate to the same | a == 2 # True |
== | True if both sides evaluate to the same | a == b # False |
!= | True if both side do not evaluate to the same | a != b # True |
!= | True if both side do not evaluate to the same | a != 3 # True |
There is also the identity operator is
, but it does something different than ==
and the explanation goes beyound the scope of this course. You can read about it here if you're curious. For now, in 99% of case you actually want ==
.
a = 2
b = 3
print(a == b)
print(a != b)
False True
This works between all types of objects:
#comparing the equality of lists
alist = [1, 2, 3]
blist = [1, 2, 3]
print(alist == blist)
#comparing dictionaries
food_dict = {
'fruit': ['banana', 'apple', 'strawberry'],
'veggies': ['eggplant', 'carrot']
}
city_population = {
'Tokyo': 13350000, # a key-value pair
'Los Angeles': 18550000,
'New York City': 8400000,
'San Francisco': 1837442,
}
print(food_dict == city_population)
# we already saw == on integers and strings above
True False
Exercise 1¶
~ 5 minutes
a. What will be the result of this comparison?
x = 2
y = "Anthony"
x < y
True
False
- Error
b. What about this comparison?
x = 12.99
y = 12
x >= y
True
False
- Error
c. And this comparison?
x = 5
y = "Hanna"
x == y
True
False
- Error
Truthiness¶
In addition to comparing objects to each other, it is important to note that all Python objects are by themselves either True
or False
. We call this concept Truthiness.
x = 1
y = 0
bool(x) # True
bool(y) # False
Empty objects such as empty lists/tuples/arrays/strings, the None
object, and the int
0
and float
0.0
are by definition False.
All non-empty objects are by definition True.
# empty list
a = []
print(bool(a))
False
# 0, '' and None are False
a = 0
b = None
c = ''
print(bool(a))
print(bool(b))
print(bool(c))
False False False
# non-empty objects are True
a = [1,2,3]
b = 5
c = 'Hi!'
print(bool(a))
print(bool(b))
print(bool(c))
Logical Operators¶
Logical operators are used in conditional statements.
and
, True if both a AND b are true (logical conjunction)or
, True if either a OR b are true (logical disjunction)not
, True if the opposite of a is true (logical negation)
# logical operator: and
# will only return true if both conditions are true!
a = True
b = True
res1 = a and b
print(res1)
True
c = False
res2 = a and c
print(res2)
False
# logical operator: or
# will return true if at least one of the conditions is true
#remember a = True and c = False as defined above
res3 = a or c
print(res3)
True
# logical operator: not
# negates: True becomes False and False becomes True
#remember a = True and c = False as defined above
res4 = not a
print(res4)
res5 = not c
print(res5)
False True
Membership operators¶
Membership operators test whether a certain element is part of a container.
Operator | Description | Example (a = [1, 2, 3] ) |
---|---|---|
in | True if value/variable is found in the sequence | 2 in a # True |
not in | True if value/variable is not found in the sequence | 5 not in a # False |
aa = ['alanine', 'glycine', 'tyrosine']
'alanine' in aa
True
Remember that strings are containers of characters, so in
works on them as well!
print(aa[1])
'gly' in aa[1]
glycine
True
my_s = "Python is great!"
'great' in my_s
Exercise 2¶
~10 minutes
a. What is the result of the following expression?
a = True
b = False
a or b
What do you need to change to make this expression evaluate to False
?
# your code here
b. What about the result of this expression? Why is this the result?
A hint: Check bool(y) and bool(x) individually.
x = 15
y = 0
bool(x or y)
# your code here
c. What is the result of the following expression?
x = 0
y = None
x < y
Can you compare x and y in a way that does not produce an error?
# your code here
d. How can you find out if the following list contains 'Copenhagen'?
capitals = ['Berlin', 'London', 'Copenhagen', 'Madrid']
# your code here
Flow of code¶
So far we have made code that flows linearly. The statements are executed one after each other like here:
my_var = 1
my_var += 10
print(my_var)
print(my_var == 11)
You can see, all lines start at the same left edge of the page. We say they are on the same indentation level.
But often when we program we want different things to happen depending on a condition:
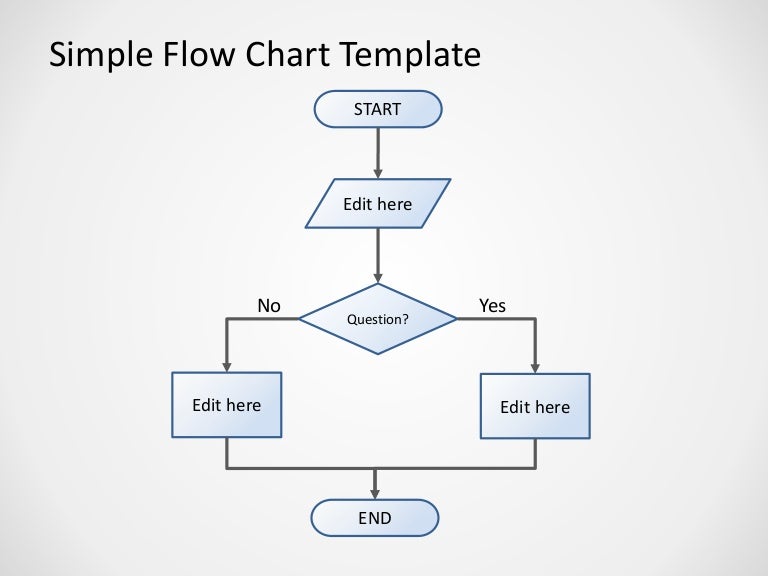
The way we control flow in python is with indentation. It defines where a line of code belongs. Lines that are on the same level of indentation will be executed linearly, one after another. Lines on a different indentation level means there is some sort of flow control happening.
if some condition is True:
do something
everything I write here is part of the if block
it will only be executed if the condition is true
more commands
#when we return to the outermost indentation level python knows the if block is over
elif some other condition is True:
do something else
else:
do something
Conditional Statements¶
Conditional statements use the keywords
if
,elif
andelse
, and they let you control which pieces of code are run based on the value of some Boolean condition.
The basic syntax of an if
block is:
if some condition is True:
do something
elif some other condition is True:
do something
else:
do something
- The lines containing
if
,elif
andelse
always need to end with a:
(colon). - Only
if
andelif
are followed by conditions, whereaselse
is never followed by anything else than a:
(colon). if
andelif
are exclusive. They cannot simultaneously be true! In fact, theelif
condition will only be checked if theif
condition is False.- Code to be executed if the condition is True always needs to be indented.
# if/else
age = 23
if age >= 18:
print("Here's your beer")
else:
print("Come back when you're 18!")
Here's your beer
The condition to be checked can also be a boolean itself! For example:
# if/else
is_weekend = True
if not is_weekend:
print("It's Monday.")
print("Go to work.")
else:
print("Sleep in and enjoy the beach.")
Sleep in and enjoy the beach.
# if/elif/else
# the elif condition will only be checked if the if condition is False
birth_year = 1950
if birth_year < 1964:
print('Ok, boomer.')
elif birth_year < 1990:
print("Sorry, the 90's was not 15 years ago. That's 2008.")
else:
print("Yeah yeah, talk to me when you're a grown-up, zoomer")
Ok, boomer.
Exercise¶
~ 5 minutes
If you set the name variable to "Gandalf" and run the script below, what will the output be? How do you get the output 'Move on then'?
name = ""
if name == "Gandalf":
print("Run, you fools!")
elif name == "Aragorn":
print("There is always hope.")
else:
print("Move on then!")
Exercise 3¶
~ 10-15 minutes
Create a variable and assign an integer as value, then build a conditional to test it:
- If the value is below 0, print "The value is negative".
- If the value is between 0 and 20 (including 0 and 20), print the value.
- Otherwise, print "Out of scope".
Test it by changing the value of the variable.